Because of my wife’s strong recommendation, I started to use Oracle Cloud to host my blog, for free 🙂
Currently, the Oracle Cloud provides four 4-core/24GB ARM64 virtual machines without any fees. This is the best part.
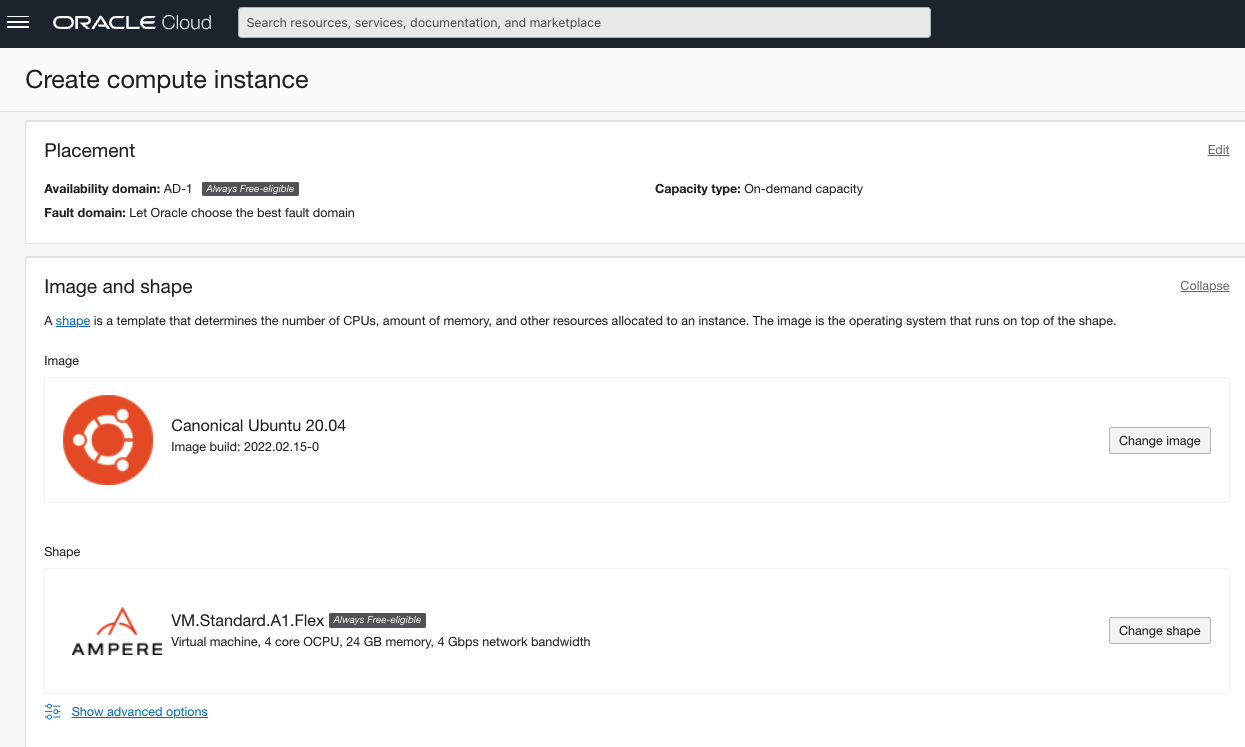
After migrating my blog from AWS (t2.micro) to this ARM64 VM, everything works well except that the permalink of my posts are all changed from date/postname
to postnumber
. (By following this link and changing my Nginx configuration file /etc/nginx/sites-available/default
, this problem has been solved just seconds ago!)
Then I started to think about whether I could deploy other services on this ARM64 VM. Here is my test code for using yolov5:
import cv2 import time import argparse import torch import torch.nn as nn IMAGE_SHAPE = (300, 300) def predict(args): img = cv2.imread(args.image_file) # detect model = torch.hub.load('ultralytics/yolov5', 'yolov5m') net = torch.load(args.classify_model, map_location=torch.device('cpu')) net.eval() softmax = nn.Softmax(dim=1) t0 = time.time() for _ in range(100): results = model(img) for img in results.render(): # Just find the most possible bird (if there are many) img = cv2.resize(img, IMAGE_SHAPE) tensor_img = torch.from_numpy(img) result = net(tensor_img.unsqueeze(0).permute(0, 3, 1, 2).float()) result = softmax(result) values, indices = torch.topk(result, 10) t1 = time.time() print(indices) print('time:', t1 - t0) if __name__ == '__main__': parser = argparse.ArgumentParser() parser.add_argument('--image_file', default="2400.jpg", type=str, help='Image file to be predicted') parser.add_argument('--classify_model', default='bird_cls_1160000.pth', type=str, help='Trained ckpt file path to open') args = parser.parse_args() predict(args)
It only cost 0.67 seconds for a yolov5m
model. This means the Ampere Arm64 core is as fast as AMD EPYC!