I found a very interesting picture:
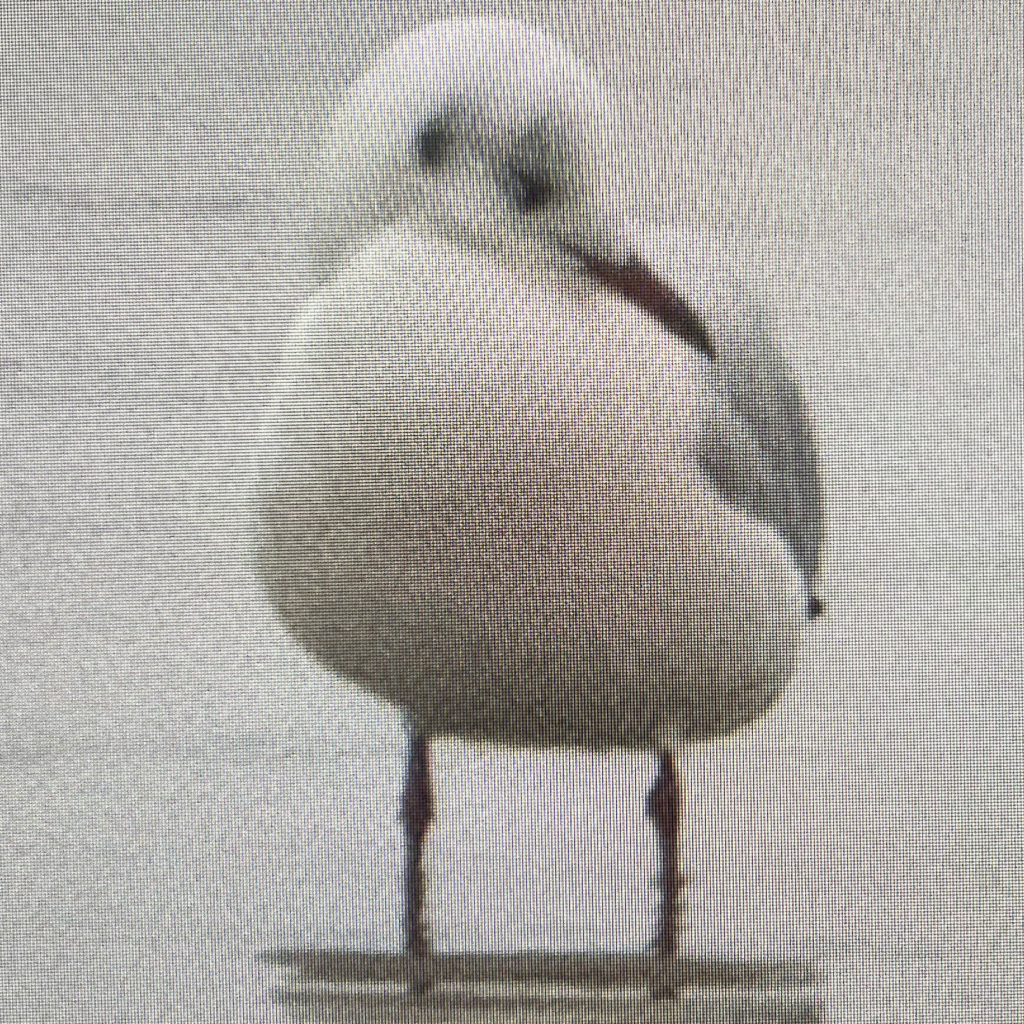
The size of this image is about 8MB although it’s blurring. Then I use below python code to try to resize it using different interpolation strategy:
import cv2 img = cv2.imread("/Users/robin/Downloads/ou1.png") for inter, name in [(cv2.INTER_AREA, "area"), (cv2.INTER_CUBIC, "cubic"), (cv2.INTER_LINEAR, "linear")]: after = cv2.resize(img, (310, 310), inter) cv2.imwrite(f"{name}.bmp", after)
“area” | “cubic” | “linear” |
![]() | ![]() | ![]() |
Only cv2.INTER_AREA works well.
Then let me try dart language:
import 'dart:io'; import 'package:image/image.dart' as img; void main() async { // Load the image final file = File('/Users/robin/Downloads/ou1.png'); final bytes = await file.readAsBytes(); final image = img.decodeImage(bytes); if (image == null) { print("Failed to load image"); return; } // Resize and save images with different methods for (var method in ['area', 'cubic', 'linear', 'average']) { img.Image resized; if (method == 'area' || method == 'linear') { // Use `copyResize` for these, `area` will behave similarly to default resized = img.copyResize(image, width: 310, height: 310); } else if (method == 'cubic') { // Simulate cubic interpolation (approximation) resized = img.copyResize(image, width: 310, height: 310, interpolation: img.Interpolation.cubic); } else if (method == 'average') { resized = img.copyResize(image, width: 310, height: 310, interpolation: img.Interpolation.average); } else { print("Unknown interpolation method: $method"); continue; } // Save the resized image final output = File('$method.bmp'); await output.writeAsBytes(img.encodePng(resized)); print("Saved resized image using $method interpolation as $method.bmp"); } }
The result looks also terrible except just one strategy “average”:
“area” | “cubic” | “linear” | “average” |
![]() | ![]() | ![]() | ![]() |
So, better use “Interpolation.average” in dart language.